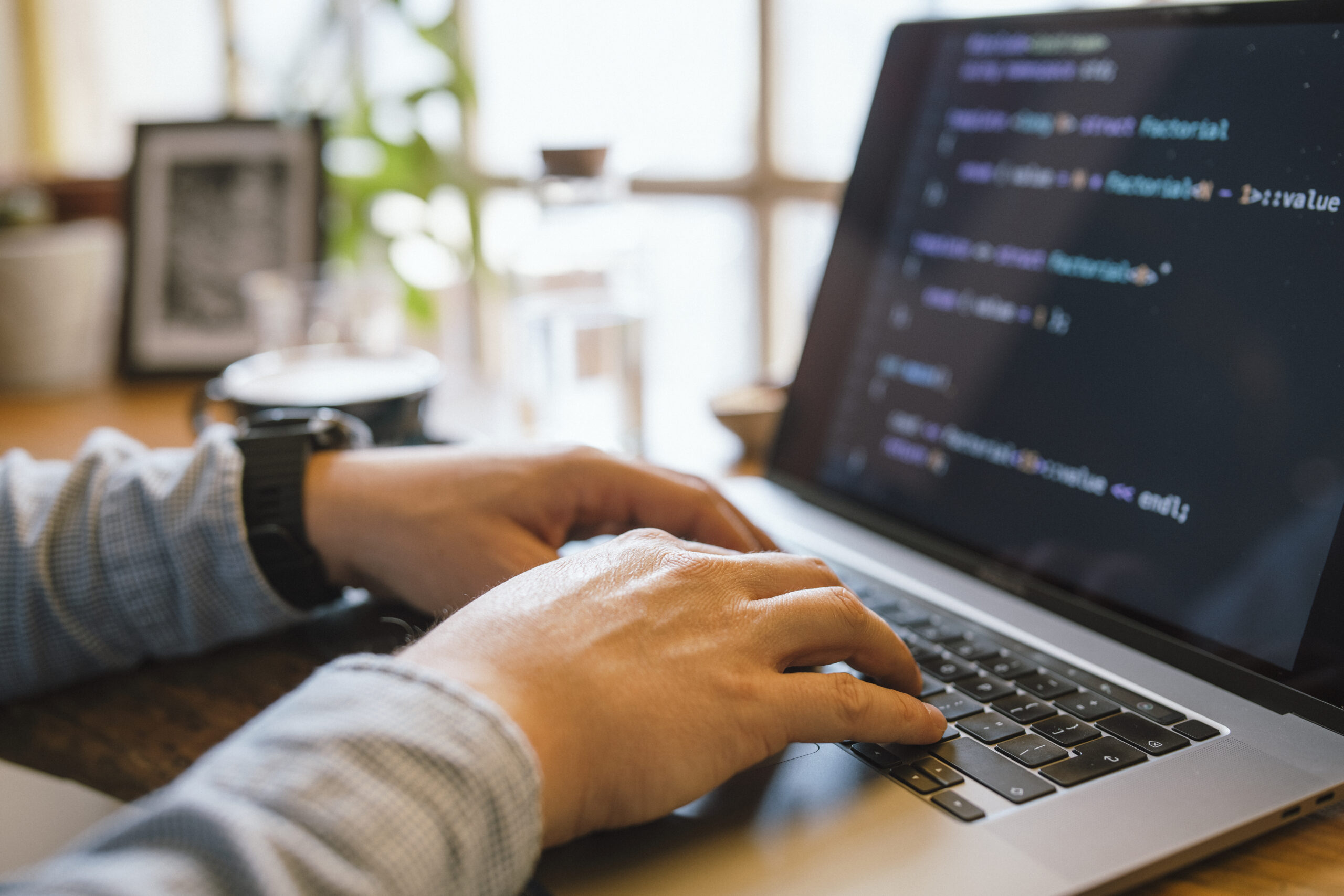
Debugging is Among the most essential — nevertheless generally missed — abilities within a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why matters go wrong, and Studying to Believe methodically to solve issues effectively. Regardless of whether you're a newbie or even a seasoned developer, sharpening your debugging expertise can conserve hours of aggravation and significantly enhance your productivity. Listed here are several strategies to help builders stage up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
One of the fastest approaches developers can elevate their debugging abilities is by mastering the tools they use everyday. When composing code is 1 part of enhancement, figuring out the best way to interact with it correctly through execution is equally important. Modern-day advancement environments come Geared up with effective debugging abilities — but a lot of developers only scratch the area of what these equipment can perform.
Consider, such as, an Integrated Development Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications let you established breakpoints, inspect the value of variables at runtime, move by way of code line by line, as well as modify code to the fly. When utilized the right way, they Allow you to notice precisely how your code behaves all through execution, which can be a must have for tracking down elusive bugs.
Browser developer equipment, such as Chrome DevTools, are indispensable for front-close developers. They enable you to inspect the DOM, monitor network requests, perspective genuine-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, sources, and community tabs can turn aggravating UI challenges into manageable duties.
For backend or process-level developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage in excess of functioning processes and memory management. Understanding these instruments may have a steeper Understanding curve but pays off when debugging effectiveness challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be relaxed with Variation control methods like Git to comprehend code heritage, come across the precise instant bugs were introduced, and isolate problematic modifications.
Eventually, mastering your equipment suggests going past default settings and shortcuts — it’s about building an intimate understanding of your advancement setting to ensure when difficulties occur, you’re not missing in the dead of night. The higher you already know your instruments, the greater time it is possible to commit fixing the actual issue instead of fumbling via the process.
Reproduce the Problem
One of the most significant — and infrequently forgotten — methods in successful debugging is reproducing the trouble. Prior to jumping into your code or building guesses, developers require to create a reliable setting or situation exactly where the bug reliably seems. With no reproducibility, fixing a bug becomes a activity of possibility, usually leading to squandered time and fragile code adjustments.
The initial step in reproducing a challenge is gathering just as much context as you can. Inquire thoughts like: What actions led to The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater detail you may have, the less difficult it results in being to isolate the exact conditions underneath which the bug occurs.
When you finally’ve collected ample data, try to recreate the situation in your local setting. This could indicate inputting the same knowledge, simulating similar consumer interactions, or mimicking process states. If the issue seems intermittently, take into consideration creating automatic tests that replicate the edge scenarios or state transitions concerned. These assessments not only assistance expose the situation but additionally reduce regressions in the future.
Often, the issue could possibly be ecosystem-certain — it'd happen only on specific functioning methods, browsers, or underneath certain configurations. Using equipment like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a step — it’s a attitude. It involves tolerance, observation, and a methodical method. But after you can persistently recreate the bug, you might be now midway to correcting it. With a reproducible situation, You should utilize your debugging applications extra effectively, test prospective fixes securely, and talk far more Plainly using your staff or end users. It turns an abstract grievance into a concrete challenge — Which’s where by developers thrive.
Study and Comprehend the Error Messages
Mistake messages in many cases are the most worthy clues a developer has when a thing goes Mistaken. As an alternative to viewing them as irritating interruptions, developers should really study to deal with error messages as immediate communications with the technique. They usually tell you what precisely took place, in which it happened, and in some cases even why it took place — if you understand how to interpret them.
Start by examining the concept cautiously As well as in entire. Numerous builders, particularly when under time tension, glance at the first line and promptly commence making assumptions. But further within the mistake stack or logs could lie the true root lead to. Don’t just copy and paste mistake messages into search engines like yahoo — read and fully grasp them initial.
Crack the error down into sections. Is it a syntax mistake, a runtime exception, or a logic error? Will it stage to a selected file and line quantity? What module or functionality activated it? These questions can information your investigation and point you toward the liable code.
It’s also useful to be aware of the terminology from the programming language or framework you’re working with. Mistake messages in languages like Python, JavaScript, or Java usually abide by predictable patterns, and Finding out to acknowledge these can significantly hasten your debugging process.
Some problems are obscure or generic, and in All those cases, it’s vital to look at the context wherein the error occurred. Check out similar log entries, input values, and recent alterations from the codebase.
Don’t overlook compiler or linter warnings either. These usually precede much larger challenges and provide hints about possible bugs.
Eventually, mistake messages are certainly not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, aiding you pinpoint troubles speedier, cut down debugging time, and turn into a more efficient and confident developer.
Use Logging Properly
Logging is Just about the most strong instruments in a very developer’s debugging toolkit. When applied correctly, it offers authentic-time insights into how an software behaves, serving to you fully grasp what’s occurring beneath the hood while not having to pause execution or phase throughout the code line by line.
A superb logging approach begins with realizing what to log and at what degree. Typical logging levels include DEBUG, Facts, Alert, Mistake, and FATAL. Use DEBUG for comprehensive diagnostic information during development, Facts for normal functions (like productive begin-ups), WARN for probable troubles that don’t split the application, Mistake for true issues, and FATAL if the technique can’t carry on.
Avoid flooding your logs with too much or irrelevant knowledge. An excessive amount of logging can obscure important messages and decelerate your program. Focus on critical functions, state improvements, input/output values, and important determination factors in your code.
Structure your log messages clearly and continually. Contain context, such as timestamps, ask for IDs, and performance names, so it’s simpler to trace issues in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting the program. They’re Primarily useful in output environments in which stepping as a result of code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about equilibrium and clarity. Using a very well-thought-out logging strategy, you could reduce the time it requires to identify issues, obtain further visibility into your purposes, and improve the General maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To efficiently establish and take care of bugs, developers should strategy the method just like a detective resolving a secret. This mindset assists break down sophisticated troubles into workable areas and observe clues logically to uncover the foundation induce.
Start by gathering evidence. Look at the signs and symptoms of the trouble: error messages, incorrect output, or efficiency troubles. Similar to a detective surveys a criminal offense scene, acquire as much pertinent details as it is possible to with no jumping to conclusions. Use logs, check instances, and user reports to piece together a transparent photograph of what’s occurring.
Following, kind hypotheses. Request oneself: What may be triggering this conduct? Have any modifications lately been made into the codebase? Has this challenge transpired just before below similar instances? The purpose is always to narrow down alternatives and establish prospective culprits.
Then, test your theories systematically. Seek to recreate the situation in the controlled natural environment. In case you suspect a specific functionality or element, isolate it and verify if The problem persists. Like a detective conducting interviews, check with your code queries and let the final results lead you nearer to the truth.
Fork out close notice to tiny details. Bugs generally hide from the least predicted locations—similar to a missing semicolon, an off-by-just one error, or maybe a race situation. Be extensive and affected person, resisting the urge to patch The problem without entirely comprehending it. Momentary fixes may conceal the actual issue, just for it to resurface afterwards.
Finally, retain notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging approach can help you save time for potential difficulties and help Other folks have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical competencies, method troubles methodically, and come to be more effective at uncovering hidden troubles in elaborate systems.
Compose Assessments
Producing checks is among the most effective methods to increase your debugging techniques and In general development efficiency. Exams not merely support capture bugs early and also function a security Web that offers you assurance when earning changes to your codebase. A nicely-tested application is simpler to debug since it lets you pinpoint just the place and when a challenge happens.
Begin with unit exams, which concentrate on person functions or modules. These small, isolated tests can immediately expose irrespective of whether a certain bit of logic is Functioning as anticipated. Whenever a test fails, you immediately know where by to glimpse, noticeably cutting down enough time invested debugging. Unit checks are In particular valuable for catching regression bugs—concerns that reappear following previously staying mounted.
Up coming, integrate integration assessments and stop-to-finish checks into your workflow. These enable be certain that numerous aspects of your software function alongside one another efficiently. They’re specifically useful for catching bugs that come about in sophisticated systems with many elements or products and services interacting. If anything breaks, your tests can inform you which A part of the pipeline unsuccessful and below what disorders.
Composing tests also forces you to think critically about your code. To check a characteristic properly, you may need to know its inputs, predicted outputs, and edge instances. This standard of comprehending Obviously leads to higher code composition and fewer bugs.
When debugging a concern, crafting a failing check that reproduces the bug is often a powerful initial step. As soon as the test fails persistently, you could give attention to repairing the bug and check out your check move when The difficulty is fixed. This method makes sure that a similar bug doesn’t return in the future.
In a nutshell, producing checks turns debugging from a aggravating guessing match right into a structured and predictable system—assisting you catch far more bugs, speedier and more reliably.
Consider Breaks
When debugging a difficult situation, it’s uncomplicated to be immersed in the problem—staring at your screen for hours, making an attempt Resolution immediately after Alternative. But one of the most underrated debugging tools is simply stepping away. Taking breaks helps you reset your brain, lessen annoyance, and infrequently see The problem from the new viewpoint.
When you are also near to the code for also extended, cognitive fatigue sets in. You could begin overlooking apparent mistakes or misreading code that you simply wrote just hours before. During this point out, your Mind turns into significantly less effective at issue-resolving. A brief stroll, a coffee break, or simply switching to a unique process for 10–15 minutes can read more refresh your aim. Quite a few builders report locating the root of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job from the track record.
Breaks also assist reduce burnout, In particular for the duration of lengthier debugging classes. Sitting down in front of a display screen, mentally caught, is not only unproductive and also draining. Stepping away allows you to return with renewed Electricity as well as a clearer mindset. You would possibly abruptly notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you in advance of.
Should you’re trapped, an excellent general guideline is usually to established a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver around, extend, or do one thing unrelated to code. It may come to feel counterintuitive, Particularly less than tight deadlines, but it surely actually brings about quicker and simpler debugging in the long run.
Briefly, taking breaks just isn't an indication of weakness—it’s a wise system. It provides your Mind House to breathe, improves your point of view, and allows you avoid the tunnel eyesight That always blocks your progress. Debugging can be a psychological puzzle, and rest is a component of resolving it.
Discover From Every single Bug
Each individual bug you come across is much more than simply A short lived setback—It is a chance to improve to be a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural concern, each can instruct you something beneficial should you make the effort to replicate and review what went wrong.
Begin by asking by yourself a few important queries as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it happen to be caught earlier with much better methods like unit screening, code testimonials, or logging? The solutions typically reveal blind spots inside your workflow or knowing and enable you to Create much better coding behaviors transferring forward.
Documenting bugs can also be an excellent habit. Keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring challenges or prevalent faults—which you could proactively stay away from.
In group environments, sharing what you've acquired from the bug using your peers is usually In particular strong. Regardless of whether it’s through a Slack information, a short write-up, or A fast information-sharing session, helping Many others stay away from the same difficulty boosts crew efficiency and cultivates a much better Understanding culture.
Far more importantly, viewing bugs as lessons shifts your way of thinking from stress to curiosity. Rather than dreading bugs, you’ll start out appreciating them as crucial parts of your growth journey. In the end, a lot of the greatest builders usually are not those who compose fantastic code, but people who consistently understand from their mistakes.
Ultimately, each bug you resolve provides a brand new layer to the talent set. So following time you squash a bug, have a second to mirror—you’ll occur away a smarter, a lot more able developer because of it.
Conclusion
Increasing your debugging abilities normally takes time, observe, and patience — even so the payoff is large. It tends to make you a more successful, confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be superior at what you do.